PyQt QLineEdit (textbox input)
The QLineEdit class is a single line text box control that can enter a single line string.
QLineEdit allows users to enter and edit single lines of plain text and provides many useful editing features, including: undo and redo, cut and paste, and drag and drop (see setDragEnabled()).
By changing the echoMode() of the input box, it can also be set to a “write only” field for entering passwords, etc.
The length of the text can be limited to maxLength(), which can be arbitrarily limited using a validator() or inputMask(). When toggling validator and input mask in the same input box, it is best to clear the validator or input mask to prevent uncertain behavior.
Book: Create Desktop Apps with Python PyQt5
QLineEdit example
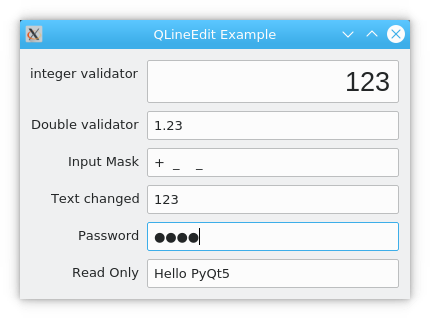
The 1st text box: shows text using custom fonts, right alignment, and allowing integer entries.
The 2nd text box: restricts entry to two decimal places.
The 3rd text box: set the mask input format and the input mask should be used for the phone number.
The 4th text box: which needs to transmit the signal textChanged, is connected to the slot function textchanged().
The 5th text box: set the display mode to Password.
The 6th text box: showing a default text that cannot be edited, is set to read-only.
from PyQt5.QtWidgets import QApplication,QLineEdit,QWidget,QFormLayout
from PyQt5.QtGui import QIntValidator,QDoubleValidator,QFont
from PyQt5.QtCore import Qt
import sys
class lineEditDemo(QWidget):
def __init__(self,parent=None):
super().__init__(parent)
e1 = QLineEdit()
e1.setValidator(QIntValidator())
e1.setMaxLength(4)
e1.setAlignment(Qt.AlignRight)
e1.setFont(QFont("Arial",20))
e2 = QLineEdit()
e2.setValidator(QDoubleValidator(0.99,99.99,2))
e3 = QLineEdit()
e3.setInputMask("+99_9999_999999")
e4 = QLineEdit()
e4.textChanged.connect(self.textchanged)
e5 = QLineEdit()
e5.setEchoMode(QLineEdit.Password)
e6 = QLineEdit("Hello PyQt5")
e6.setReadOnly(True)
e5.editingFinished.connect(self.enterPress)
flo = QFormLayout()
flo.addRow("integer validator",e1)
flo.addRow("Double validator",e2)
flo.addRow("Input Mask",e3)
flo.addRow("Text changed",e4)
flo.addRow("Password",e5)
flo.addRow("Read Only",e6)
self.setLayout(flo)
self.setWindowTitle("QLineEdit Example")
def textchanged(self,text):
print("Changed: " + text)
def enterPress(self):
print("Enter pressed")
if __name__ == "__main__":
app = QApplication(sys.argv)
win = lineEditDemo()
win.show()
sys.exit(app.exec_())