QListWidget
The QListWidget class is a versatile tool in the PyQt5 toolkit, providing an interface for displaying and manipulating a list of items. Each item in this list is represented by a QListWidgetItem object. Furthermore, users can configure the QListWidget to support multiple item selection at once.
Book: Create Desktop Apps with Python PyQt5.
Utilizing QListWidget in PyQt5
The following example demonstrates how to effectively use the QListWidget
. Within this example, multiple items are added to the list using the addItem()
method.
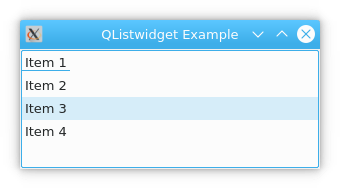
When an item in the widget is selected, a popup will appear. This interaction is facilitated by a clicked event, which triggers a QMessageBox to be displayed.
import sys
from PyQt5.QtCore import *
from PyQt5.QtGui import *
from PyQt5.QtWidgets import *
class ListWidgetDemo(QListWidget):
def onItemClicked(self, item):
QMessageBox.information(self, "QListWidget Interaction", "You selected: " + item.text())
if __name__ == '__main__':
app = QApplication(sys.argv)
demoListWidget = ListWidgetDemo()
demoListWidget.resize(300, 120)
demoListWidget.addItem("Option 1")
demoListWidget.addItem("Option 2")
demoListWidget.addItem("Option 3")
demoListWidget.addItem("Option 4")
demoListWidget.setWindowTitle('QListWidget Demonstration')
demoListWidget.itemClicked.connect(demoListWidget.onItemClicked)
demoListWidget.show()
sys.exit(app.exec_())
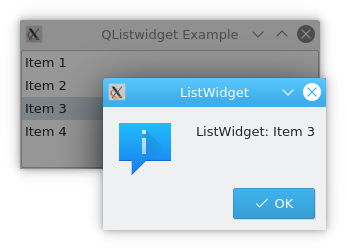
It’s worth noting that this example draws parallels with the QListView example. The key distinction lies in the controls; one uses QListView while the other employs QListWidget.
Commonly used methods for QListWidget
include:
addItem()
: Adds a single item.addItems()
: Adds multiple items.insertItem()
: Inserts an item at a specific position.clear()
: Clears all items.setCurrentItem()
: Sets the current item.sortItems()
: Sorts the items.
Events associated with QListWidget
are:
currentItemChanged
: Triggered when the current item changes.itemClicked
: Triggered when an item is clicked.
Drag and Drop with QListWidget
The QListWidget class also supports drag and drop functionality. In the following example, items can be dragged from a QListWidget on the right and dropped onto a QListWidget on the left.
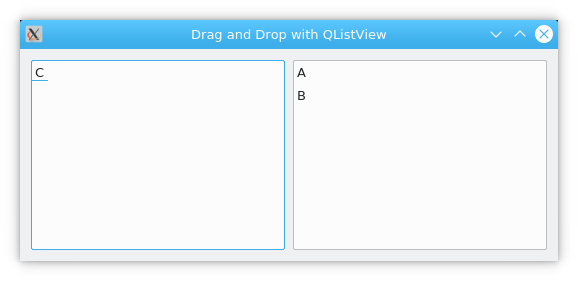
import sys
from PyQt5.QtGui import *
from PyQt5.QtCore import *
from PyQt5.QtWidgets import *
class CustomDropList(QListWidget):
def __init__(self):
super(CustomDropList, self).__init__()
self.setAcceptDrops(True)
def handleDropEvent(self, dropEvent):
sourceList = dropEvent.source()
selectedItems = sourceList.selectedItems()
for item in selectedItems:
sourceList.takeItem(sourceList.indexFromItem(item).row())
self.addItem(item)
print('Completed Drop Operation')
class DragDropDemo(QWidget):
def __init__(self):
super(DragDropDemo, self).__init__()
self.setWindowTitle('Drag and Drop QListWidget Demo')
layout = QHBoxLayout()
self.leftList = CustomDropList()
self.rightList = QListWidget()
initialItems = ['Item A', 'Item B', 'Item C']
self.rightList.addItems(initialItems)
self.rightList.setDragEnabled(True)
self.rightList.setDragDropOverwriteMode(False)
self.rightList.setSelectionMode(QAbstractItemView.ExtendedSelection)
self.rightList.setDefaultDropAction(Qt.MoveAction)
layout.addWidget(self.leftList)
layout.addWidget(self.rightList)
self.setLayout(layout)
if __name__ == '__main__':
app = QApplication(sys.argv)
demoWidget = DragDropDemo()
demoWidget.show()
sys.exit(app.exec_())