pyqt tooltip
The PyQt5 library offers a powerful feature called tooltips, which provide hints to users while using a desktop application.
A tooltip can be thought of as a helpful message that pops up when a user hovers over a particular element in a user interface.
Book: Create Desktop Apps with Python PyQt5
Utilizing Tooltips in PyQt
When you hover your mouse pointer over specific elements in a PyQt5 application, such as a button, a small message (tooltip) will appear to provide more information or guidance to the user.
import sys
from PyQt5.QtWidgets import (QApplication, QWidget, QToolTip, QPushButton)
from PyQt5.QtGui import QFont
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
QToolTip.setFont(QFont('Arial', 14))
self.setToolTip('Tooltip for <b>QWidget</b>')
btn = QPushButton('Button', self)
btn.setToolTip('Tooltip for <b>QPushButton</b>')
btn.resize(btn.sizeHint())
btn.move(50, 50)
self.setGeometry(300, 300, 300, 220)
self.setWindowTitle('PyQt Tooltip Demo')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
This code illustrates the process of adding tooltips to different PyQt5 controls:
QToolTip.setFont(QFont('Arial', 14))
- This line defines the appearance of the tooltip. Here, we’ve chosen the Arial font at a 14px size. This ensures the tooltip is clearly readable.self.setToolTip('Tooltip for <b>QWidget</b>')
- This method assigns a tooltip to the entire window or the QWidget. The tooltip text supports rich text format, allowing you to bold or italicize words for emphasis.The
QPushButton
is initialized, and its tooltip is set usingbtn.setToolTip('Tooltip for <b>QPushButton</b>')
. This provides contextual information about the button.btn.resize(btn.sizeHint())
andbtn.move(50, 50)
- These methods help in determining the size and position of the button on the window.
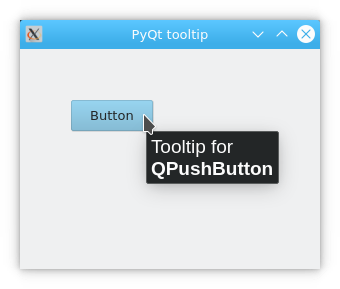