PyQt message box
This article mainly introduces the PyQt5 daily pop-up message box in detail.
By default, a QWidget closes if we click the X button on the title bar. Sometimes, we need to change this default behavior.
For example, if we have a file open in the editor, we can first display a message box that confirms the action to open or not.
Book: Create Desktop Apps with Python PyQt5
PyQt Message Box example
A simple message box is shown when the user closes the window.
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QMessageBox
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 300, 220)
self.setWindowTitle('Hello World')
self.show()
def closeEvent(self, event):
reply = QMessageBox.question(self, 'Quit', 'Are you sure you want to quit?',
QMessageBox.Yes | QMessageBox.No, QMessageBox.No)
if reply == QMessageBox.Yes:
event.accept()
else:
event.ignore()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
If we close the QWidget control, the QCloseEvent event will be generated. To modify the control properties we need to re-implement the closeEvent() event handler.
reply = QMessageBox.question(self, 'Quit', 'Are you sure you want to quit?',
QMessageBox.Yes | QMessageBox.No, QMessageBox.No)
We display a message box with two buttons, Yes and No.
- The first string appears in the title bar.
- The second string is the message text displayed in the dialog box.
- The third parameter specifies the combination of buttons in the dialog box that pops up.
- The last parameter is the default button, which is the button with the initial keyboard focus.
The return value is stored in the reply variable.
if reply == QMessageBox.Yes:
event.accept()
else:
event.ignore()
Here we test the return value using if
else
. If we click the Yes button, we accept the closure of the button control and perform an application termination event. Otherwise, we ignore the shutdown event.
After the program executes, click on the X in the upper right corner to bring up the confirmation exit message box
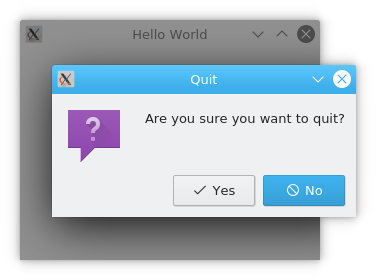