How to install PyQt5 in PyCharm
PyQt5 is a toolkit for creating Python GUI applications. As a cross-platform toolkit, PyQt can run on all major operating systems (Unix, Windows (Mac).
This article describes how to install Python + PyCharm + PyQt5.
With PyQt5, the GUI is designed in two ways:
- directly using the code to design the interface
- using QtDesigner to visualize the design, and then convert the resulting .ui file into a .py file.
Related course: Create Desktop Apps with Python PyQt5
Install Python + PyCharm + PyQt5.
Step 1. Install Python.
Visit the official website at https://www.python.org/ to download and install your target Python version.
Install PyQt5
Enter the cmd interface. Run the command and wait a moment.
pip install pyqt5 pyqt5-tools
The command is executed and PyQt5 is installed.
Test if pqyt5 is actually installed
Create a new file, example.py, and enter the following code.
import sys
from PyQt5 import QtWidgets, QtCore
app = QtWidgets.QApplication(sys.argv)
widget = QtWidgets.QWidget()
widget.resize(400, 200)
widget.setWindowTitle("This is PyQt Widget example")
widget.show()
exit(app.exec_())
After running, the following screen will pop up, indicating that PyQt is working normally.
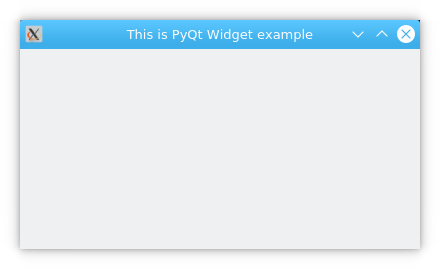
3. Install PyCharm.
Visit the official website http://www.jetbrains.com/pycharm/
Choose download and install Community version.
PyCharm Basic Configuration.
Step 1 Create a new project
Step 2 Set the default PyCharm parser.
Select File | Settings | Project: first | Project Interpreter, set Project Interpreter to The version of python you are using
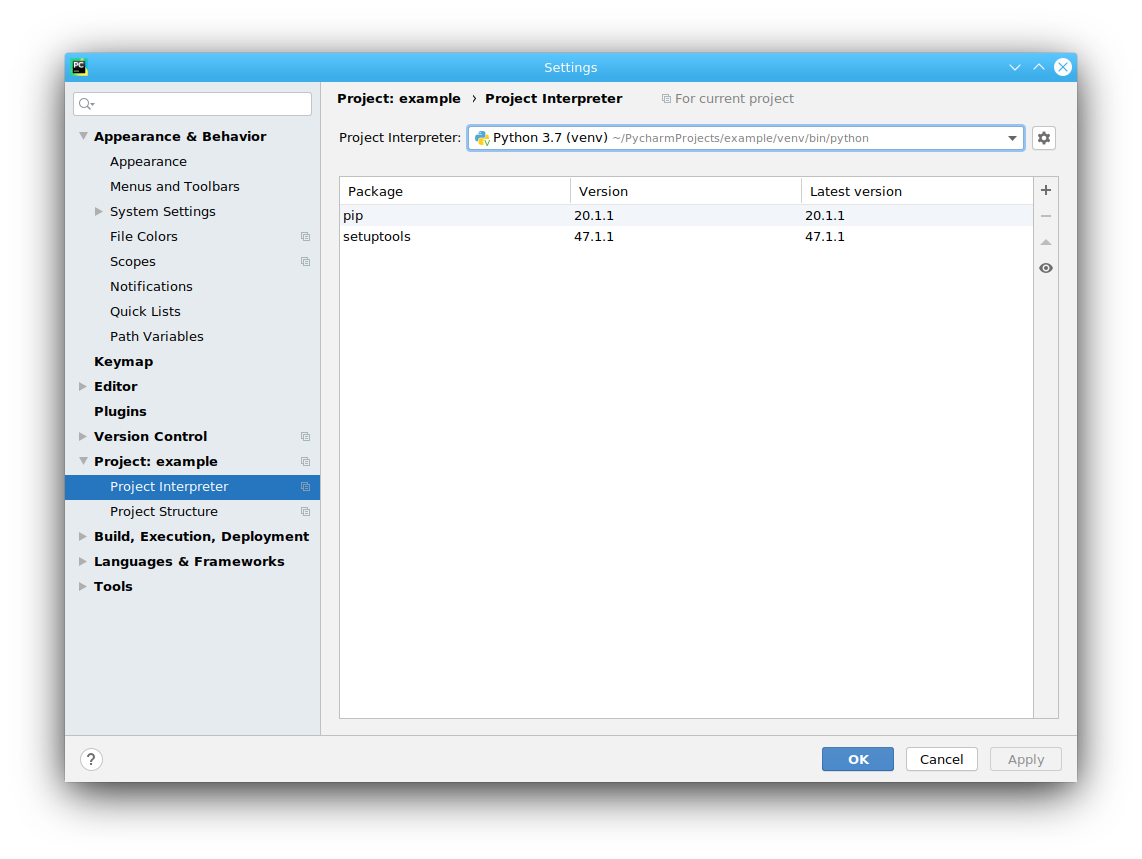
Step 3 Adding third-party libraries
Stay in the Project Interpreter interface, click on the +, find and install pyqt5. pyqt5-sip, pyqt5-tools. After successful installation, the interface should look like this.
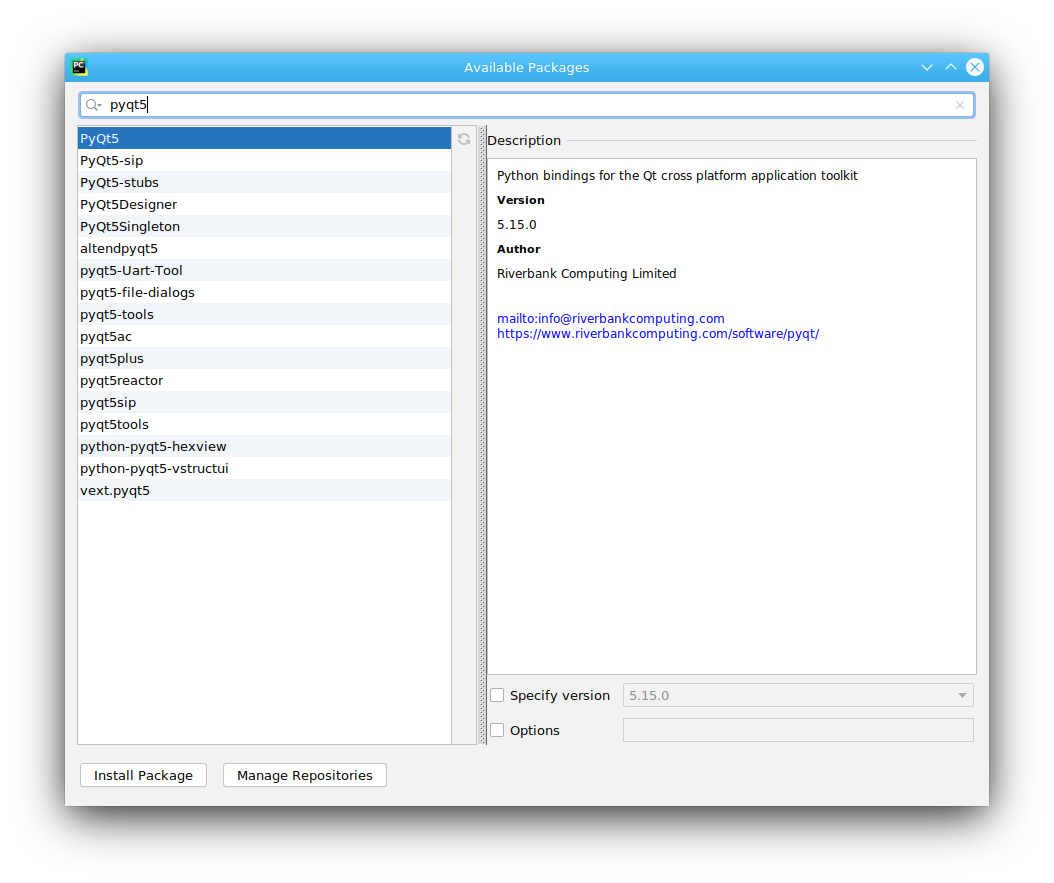
Step 4 Configuring QtDesigner
Install designer
You can start designer directly from PyCharm. Make sure designer is installed first. Designer is not installed with the pip installation.
On Fedora Linux you can do this:
sudo dnf install qt5-designer
Ubuntu Linux users can do thiS:
sudo apt-get install qttools5-dev-tools
You can also do this:
pip install pyqt5-tools
Once qt5-designer is installed, you can configure it in PyCharm.
PyCharm select File | Settings | Tools | PyCharm. External Tools, click + New Tools, Create QTdesigner and PyUIC tools
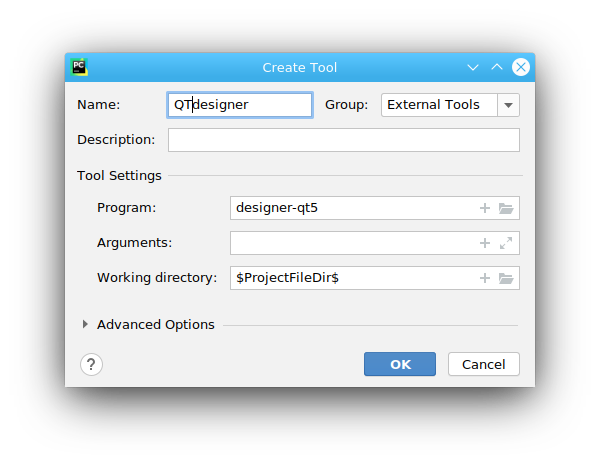
Configure two key parameters.
Program: the path to your own
designer
.Windows users can link to
designer.exe
, for exampleC:\\xxxx\AppData\Local\Programs\Python\Python35-32\\Lib\site-packages\pyqt5-tools\designer.exe
Fedora Linux users can link
designer-qt5
Ubuntu users can set it to
/usr/lib/x86_64-linux-gnu/qt5/bin/designer
Working directory:
$ProjectFileDir$
Step 5: Configure PyUIC
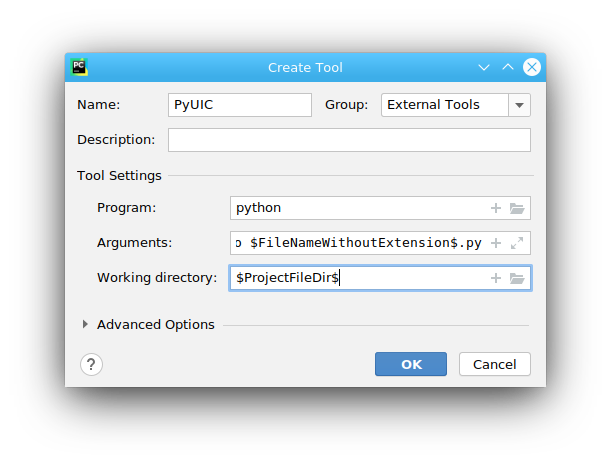
Configure three key parameters.
Program: Your own
python.exe
path For example,C:\\xxxx\AppData\\Local\Programs\Python\Python35-32\python
.Arguments:
-m PyQt5.uic.pyuic $FileName$ -o $FileNameWithoutExtension$.py
Working directory:
$ProjectFileDir$
You may like: Create Desktop Apps with Python PyQt5
Test PyQt installation
From code
Now that everything is setup, it’s time to test if everything is setup correctly.
Create a GUI interface using the code below (or any PyQt code).
from PyQt5 import QtWidgets # import PyQt5 widgets
import sys
# Create the application object
app = QtWidgets.QApplication(sys.argv)
# Create the form object
first_window = QtWidgets.QWidget()
# Set window size
first_window.resize(400, 300)
# Set the form title
first_window.setWindowTitle("The first pyqt program")
# Show form
first_window.show()
# Run the program
sys.exit(app.exec())
Select Run->Run first. You should see a PyQt window popup.
QTDesigner
Use QTDesigner to generate a GUI interface and convert it to a .py file using PyUIC.
Select Tools->ExternalTools->QTdesigner.
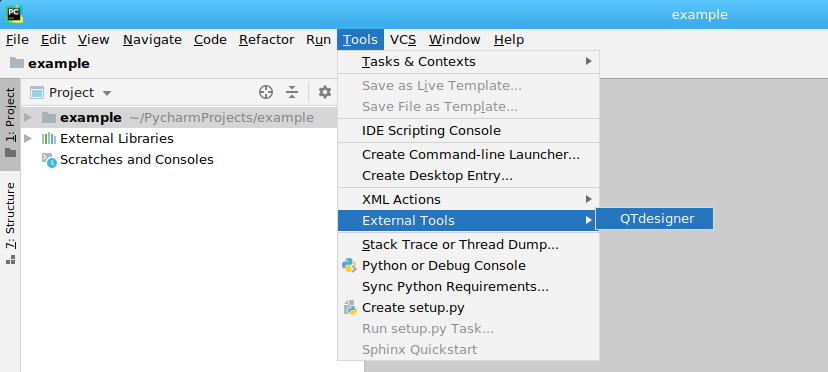
In the QT Designer interface, a GUI creation interface will pop up for the first time, click on create to automatically generate a GUI interface.
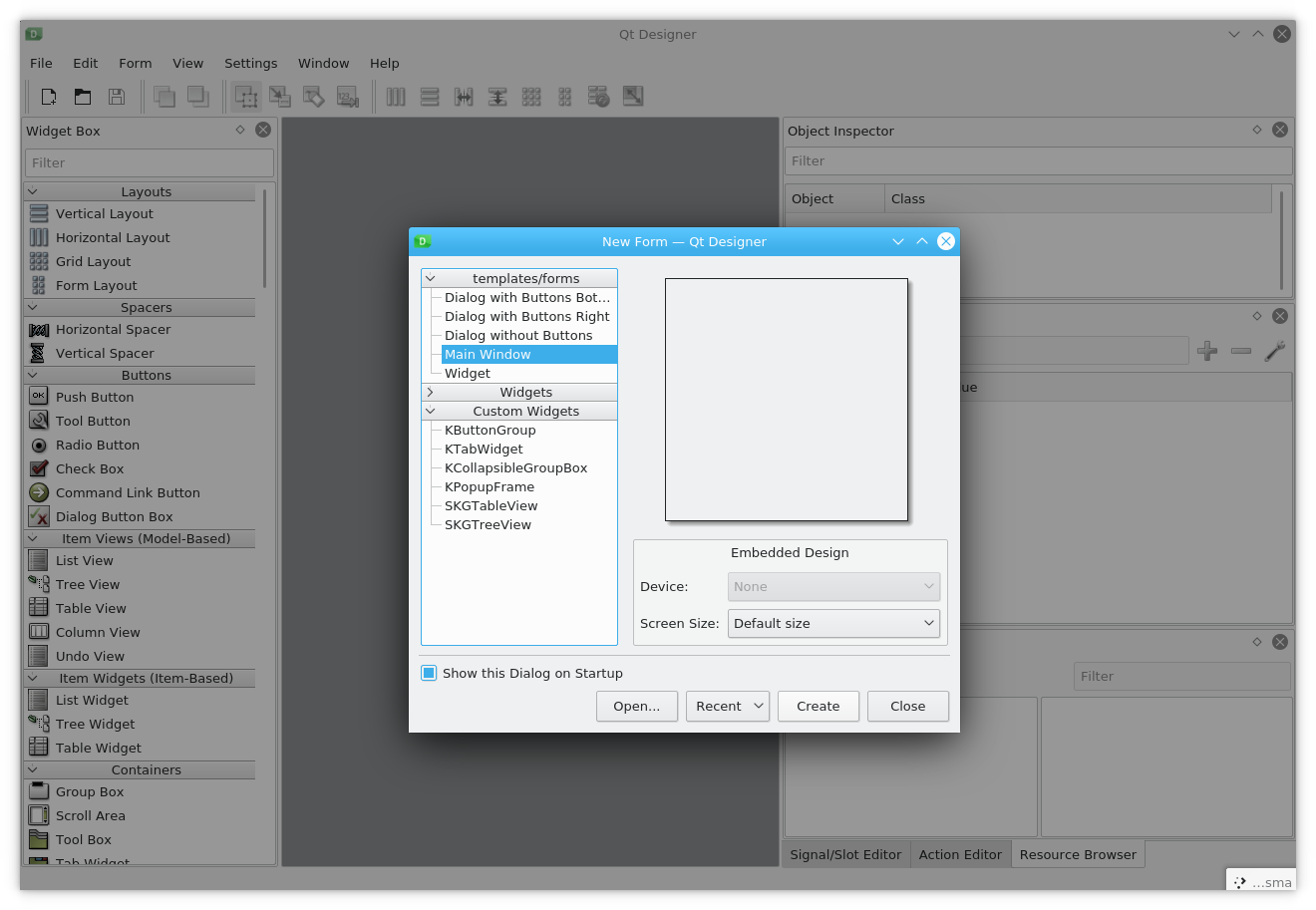
Save the interface as Untitled.ui file.
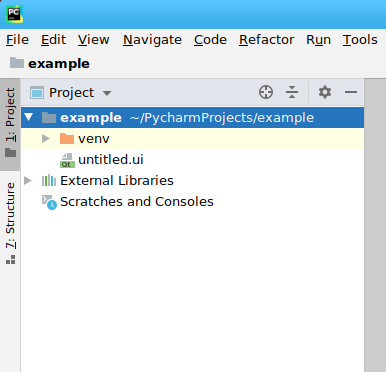
Back to the PyCharm project interface, there is an extra .ui file under the project list.
Right-click on Untitled.ui and select ExternalTools->PyUIC in the pop-up list.
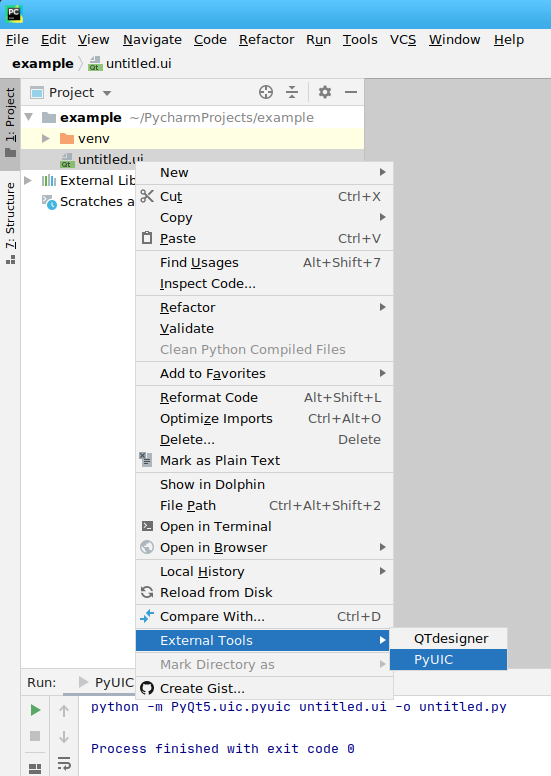
This will generate a Untitled.py file for Untitled.ui.